When it comes to designing PDFs via Visualforce pages in Salesforce, there are multiple restrictions the developers face. As soon as we add the renderAs=’PDF’ attribute, the design gets messed up. There is a reason for that: As per the official documentation, the Salesforce PDF rendering service only allows the CSS to be version 2.1 at max. Plus, the PDF version is 1.4, and the JavaScript-rendered content is not supported. Not just that but the whole Salesforce Lightning Design System (SLDS) also doesn’t work on the VF page when rendered as PDF. Hence, we are left with a limited set of options for designing PDFs. Limited but also adequate. In this post, I will guide you about the custom implementation of Grid System using CSS in VF page with renderAs PDF attribute.
The Grid System will not only make your life easier but will also speed up your development process. It will also guarantee less code when compared to other positioning techniques like using HTML tables. Before we begin, let’s discuss what is meant by Grid System.
What is Grid System?
In essence, a Grid System is a collection of rows and columns that allows the developers to easily align and position HTML elements. Usually, Grid Systems are fully responsive and give the users full control of the size of rows and columns. The nesting of rows inside rows and columns inside columns is also available. Examples would be Bootstrap and SLDS Grid.
Problem
Unable to use CSS Grid and SLDS Grid when rendering a PDF via Visualforce page in Salesforce.
Solution
Following is my custom CSS Grid implementation without using CSS Grid or Flex properties as both don’t work with PDF rendering.
Using Float for Grid System
Luckily, we can use the Float property of CSS to implement a fully working Grid System for Visualforce PDFs. Not only float is a very powerful option but it is also very common to use and has tons of resources and discussion material to fully understand its working.
The key is to make a row of 100% width and then make columns of the desired width as per the number of columns needed in a row. All the columns should be floating to the left so that they get aligned horizontally just like in a normal Grid System.
Since we are rendering as a PDF so we don’t need to worry about the responsiveness of the rows and columns.
CSS
/* Begin Custom Grid System */ .clearfix { clear: both; } .row { display: block; } .col { float: left; } .size-1-of-2 { width: 48%; } .size-1-of-3 { width: 33.33%; } .size-1-of-4 { width: 25%; } .size-1-of-5 { width: 20%; } /* End Custom Grid System */
HTML
<!-- A row with 3 eqaully sized columns --> <div class='row'> <div class='col size-1-of-3'>Column 1</div> <div class='col size-1-of-3'>Column 2</div> <div class='col size-1-of-3'>Column 3</div> <div class='clearfix'></div> </div> <!-- A row with 2 eqaully sized columns --> <div class='row'> <div class='col size-1-of-2'>Column 1</div> <div class='col size-1-of-2'>Column 2</div> <div class='clearfix'></div> </div>
Result
Following would be the output PDF.
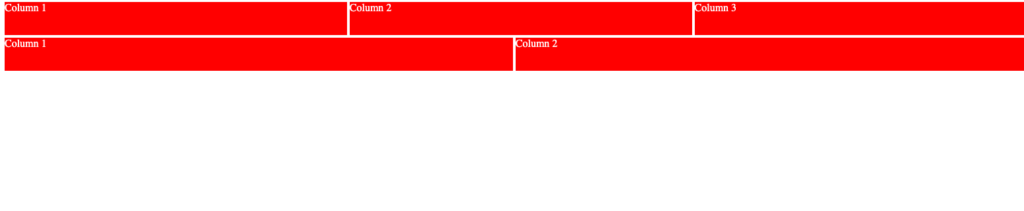
Clearfix
You might have noticed the clearfix class above. Let me explain. CSS float though powerful, yet comes with some flaws. Such as, it is a common problem with using CSS float that the elements that are floated tend to escape the regular flow of the DOM i.e. seems to come outside of their parent elements instead of staying inside as intended. For example, a child floated div would seem to come out of the parent div, and the parent div would seem to shrink. To avoid this problem, we use CSS clear. In simple words, it puts the floated elements back into their places and the parent’s size remains unaffected.
If your parent divs are only empty containers without any styling, you might not need to use a clearfix div.
Conclusion
In Visualforce pages, when we render as PDF, we lose most of the standard design features of Salesforce and CSS. One of which is the Grid System. However, by using float we can implement our own custom Grid System. A simple yet useful solution is provided above to implement a custom Grid System using CSS for Visualforce PDFs. Not only will it help you with structuring the layout but will ensure less and clean code.
Resources
- Bootstrap Grid System
- Visualforce PDF Rendering Considerations and Limitations
- SLDS Grid
- What is a Clearfix?
Don’t forget to check out the recent guide for converting Country Codes to Country Names for Data Import Wizard. For weekly Salesforce guides, keep visiting.
Also Read
- How to add cross-object fields as merge fields in Lightning Email Template
- How to generate Excel file in Aura Component of Salesforce
- How to use Lookup field in Web To Lead form in Salesforce